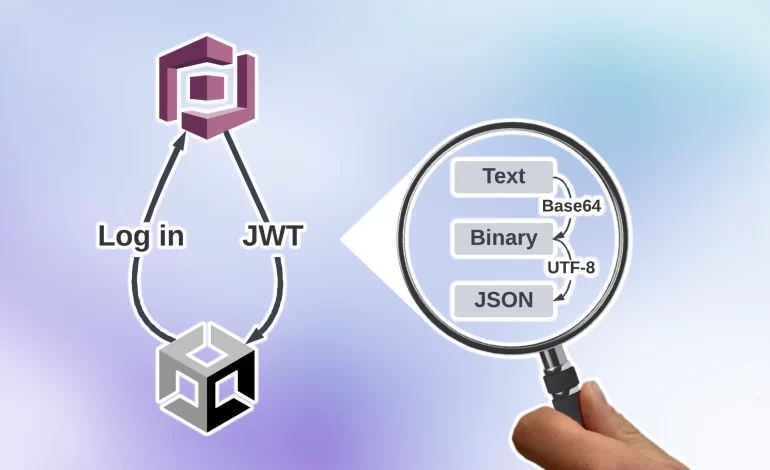
Performing Base64 Decoding on Cognito Tokens in Unity.
You may prefer watching a video instead of reading, here it is!
Weeks ago, I published a course on Udemy explaining how Unity and Amazon Cognito can work together. In one of the lectures of the course, I mentioned that reading Cognito tokens in Unity brings some advantages: since important information is contained in those tokens, we can get rid of a Cognito API call to retrieve user information. The counterpart would be that it could be somehow challenging to decode JWTs in Unity. But we love challenges so let’s do it!
If you want to know how to use Amazon Cognito as a user directory or if you want to connect Unity with Amazon services such as Lambda, S3, or DynamoDB, I recommend you check my new course on Udemy:

That said, let’s start!
Cognito works with JSON web tokens (or JWTs). After logging in with a Cognito user, Cognito sends 3 JSON web tokens to the Unity client: access, ID and refresh tokens. Those tokens contain important information that can be extracted and used by the Unity client. In this post, we will focus on the ID token, since it contains interesting information about the user.

In order to create those tokens, Cognito first converts the original JSON data into binary data and then into text data thanks to the base64 algorithm. Just a side comment about a common mistake: JSON web tokens are NOT encrypted; they are encoded, meaning that they can be easily decoded on the client side. Plenty of online tools such as jwt.io offer JWT decoding.
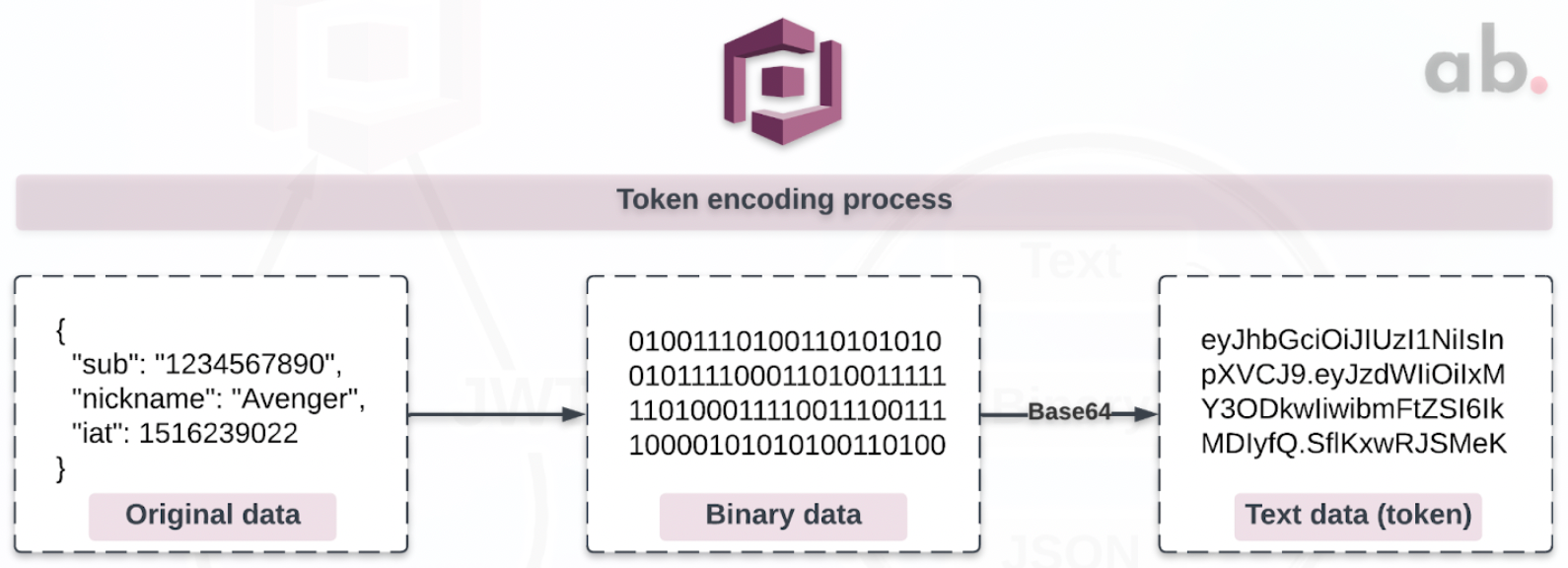
To decode JSON web tokens in Unity, we are going to do the reverse process: convert the text data into binary data and then into a string thanks to UTF-8. We could also use ASCII but I recommend using UTF-8 to handle a large range of characters: Russian, Chinese, etc.
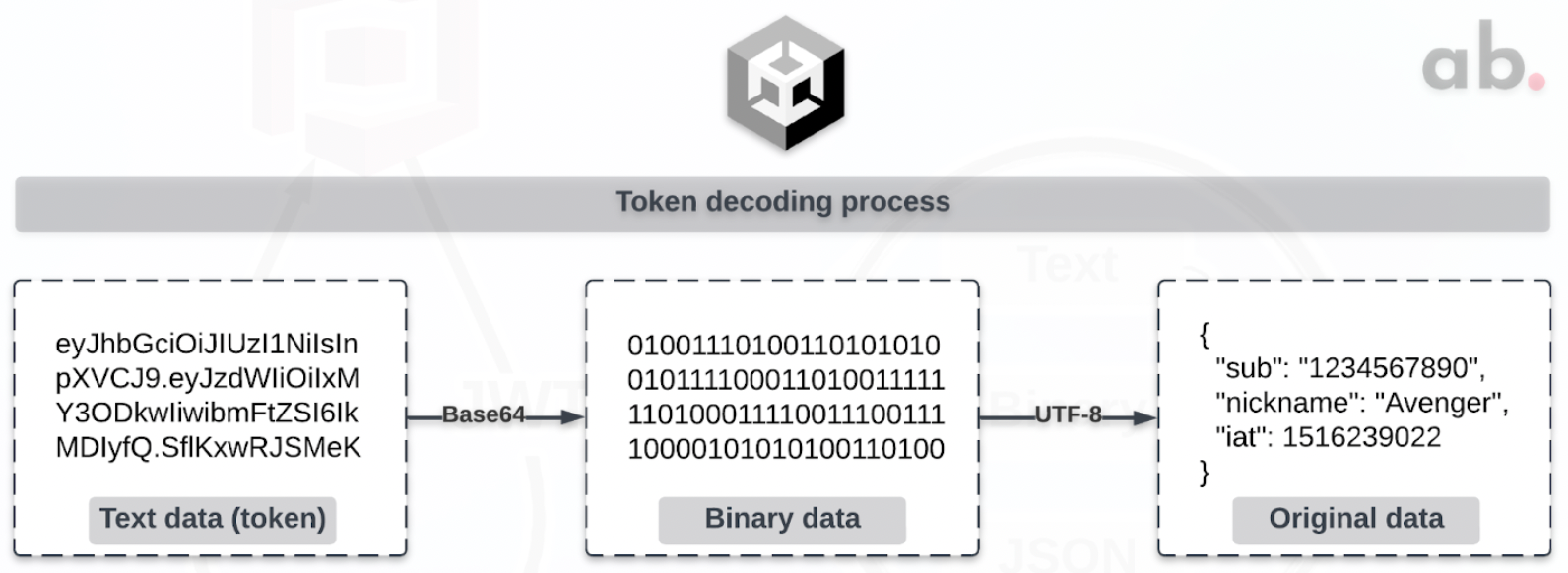
Now, let’s talk about the padding characters. When it comes to base64 decoding, the length of the data text must be a multiple of four. I won’t enter into details because that’s something complex with octets and sextets but anyway! If the length of the data text is not a multiple of four, we must add the necessary padding characters at the end. The padding character in base64 is always the equals sign (=).
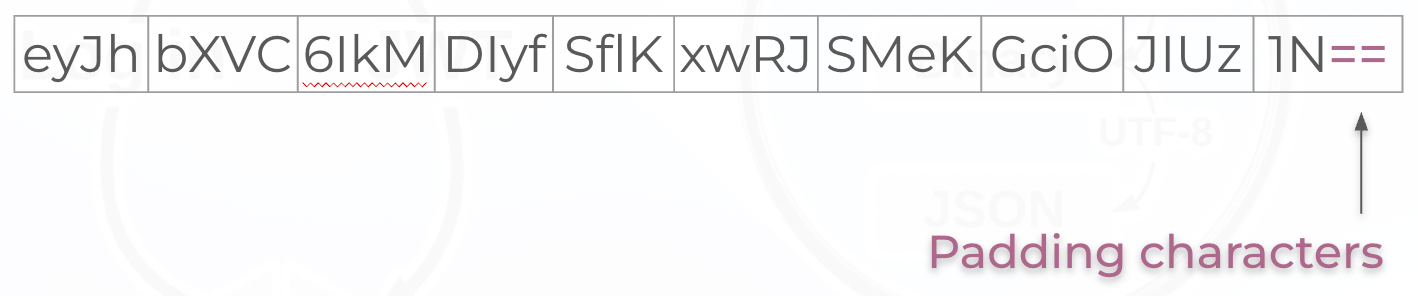
And here is the code:
Notes:
- JWTs consist of three parts separated by dots: header, payload, and signature. So, we will split the ID token thanks to the Split function (9) and we ensure that it contains three parts (11). Otherwise, this is not a valid token.
- The information we need is contained in the payload, so we isolate it (13).
- We calculate how many padding characters we will add at the end of the payload. So first, we calculate the remainder of the division by four using the modulo operator (14).
- If the remainder is equal to zero, we can decode the text. Otherwise, we calculate how many characters are missing (18) and we add the padding characters at the end of the payload (19).
- We convert the data into binary (22) thanks to the FromBase64String function and into string thanks to the GetString function (23).
- The resulting string is a JSON string, so we convert it into an object (25) and we show it on screen (26).
That’s it! This post was a bit more technical than other posts of mine but you can now decode Cognito tokens and more generally JSON web tokens in Unity.
Thanks for reading until the end! If you have any feedback or suggestions, please reach out to me on my social networks: